Whats Vite?
Vite is a bundler orchestrator that help us to manage small to medium scale web application. It’s easier to config than other bundlers and a good way to introduce yourself to the world of front end infrastructure.
Why Write Plugins?
As with any technology, sometimes we need some customization of our code and technology. In this case, we’ll want to write vite plugins in many cases where we want to change the way that our bundler works, when we want to apply different behavior on build time or when we want to change our code base and how it behaves in different environments.
Let’s start
You can write your Vite plugin inline just to get an idea of how a plugin look like. Here’s few of the plugin api options we have:
function myPlugin(): Plugin {
return {
name: "examples of plugin api",
transform: () => {
console.log("transform!");
}
}
List of Vite Plugins hooks
buildEnd
buildStart
closeWatcher
load
moduleParsed
options
resolveDynamicImport
resolveId
shouldTransformCachedModule
transform
watchChange
Vite (rollup) plugin context
When we use the hooks we’ll have few utility functions that help us write our vite plugins.
this.addWatchFile — in case we want to watch specific files
this.emitFile — emit a file output that can be used in our final bundle
this.error — error that will abort the building process
this.getCombinedSourcemap — get the previous source map of our files.
this.getFileName — get file name or chunk
this.getModuleIds — provide access to all modules ids
this.getModuleInfo — return the module information (id, code, isEntry, meta, isIncluded)
this.getWatchFiles — get files which has been watched.
this.load — load and parses the module of corresponding module id.
this.meta — contain meta data of rollup
this.parse — Use Rollup’s internal acorn instance to parse code to an AST.
this.resolve — doing a resolve of imports in our code base to module ids
this.setAssetSource — set the deferred asset resource
Our First Vite Plugin
In the next example, we’ll write a simple plugin that will help us understand how finalie version of vite config might look like. Since when we write our vite configuration, it might be very dynamic and consist of different sources of configuration. So the next plugin, will simply output the current vite config.
(function viteshowconfig() {
return {
name: "show-config",
config(config) {
console.log("config", JSON.stringify(config, null, 4));
},
};
})()
A simple function that returns an object with “name” and “config” function. The config function have the final config of vite and inside we’ll use javascript’s console.log to output the config with the help of JSON stringify and some prettification tricks to make the config readable in the terminal.
Common Vite Plugins
Here’s a list of common Vite plugins, and here’s a list of community vite plugins.
vite-ts-quick — Vue 3 + Vuex + Vue-router + TypeScript Quick Template.
vue-component-template — A template for creating own Vue3 TSX component.
vite-reactts-antd-starter — React, TypeScript, Antd.
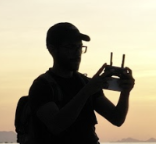
Lior Amsalem embarked on his software engineering journey in the early 2000s, delving into Pascal with a keen interest in creating, developing, and bringing new innovations to life. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply