Learn how to edit source code of files as part of the Vite plugin transpiler process. Modify them as you wish in this case to remove console log before production build
We already have another tutorial that explain how to write a vite plugin, Here I want us to write a real case of vite plugin. But before we continue, let’s answer the question what Vite is? Vite is a build tool designed for modern web development, offering a fast development server and optimized build process. It excels in providing a quick and efficient development experience, especially for Vue.js projects, but can be used with other JavaScript frameworks. Vite’s key features include a fast development server with hot module replacement (HMR) for real-time updates, optimized builds leveraging native ES Module (ESM) support in browsers for faster build times, and a plugin system for extending functionality. Overall, Vite aims to enhance the development process by leveraging modern browser features and optimizations.
Vite Plugin Remove Console log
In this practical guide I want us to write a vite plugin that remove all console.log when we build our project.
let’s start a vite plugin project from zero
yarn create vite vite-plugin-remove-console-log --template react-ts
Above code will create a folder called “vite-plugin-remove-console-log” with the template of react (meaning, vite will provide some basic react web app) and typescript, however you can use whatever template you want. note that if ‘yarn’ is not installed on your machine you can start a vite project with npm create as well! if you ave problems at this step, feel free to go over original vite documentation right here on how to start a vite project.
afterwards you should write the following commands:
cd vite-plugin-remove-console-log
yarn install
now we have a basic project with all dependencies installed. before we continue let’s write “yarn build” to create a “dist” folder with our output JS files that can be found at:
dist/assets/index-<your hash>.js
your hash in the name of the file might be different but could be look like something like that: “C5b8plLX”. open that file and see that you don’t have any console.log in the production output files (now we want to add the console log and afterward create the plugin that removes the console log).
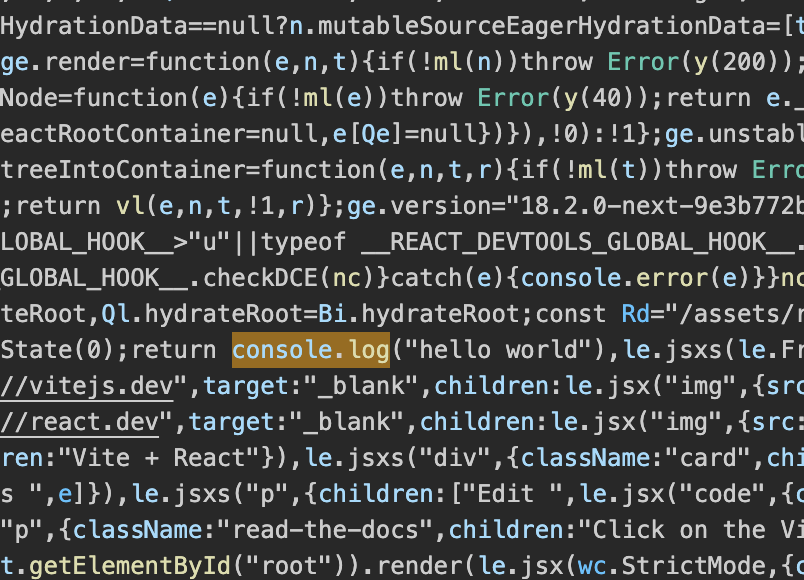
Let’s open “src/App.tsx” and edit line 8 and add the following console log:
console.log('hello world');
now let’s run again “yarn build” and open the new javascript file inside assets folder and search for the console log:
// src/App.tsx
function App() {
const [count, setCount] = useState(0)
console.log('hello world!');
return (
<>
<div>
<a href="https://vitejs.dev" target="_blank">
<img src={viteLogo} className="logo" alt="Vite logo" />
</a>
Basic Vite Config
Before we continue with our plugin let’s take a look at the basic vite config created as aprt of our new project:
// vite.config.ts
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
// https://vitejs.dev/config/
export default defineConfig({
plugins: [react()],
})
as you can see the plugins list contain a “react” plugin because we start our template of vite with react. To that configuration we’ll add our new (locally developt vite plugin that will remove all console.logs mention in our code before it gets to production)
// vite.config.ts
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
import removeConsole from './src/vite-plugins/vite-plugin-remove-console'; // <-- we develop locally so plugin is local
// https://vitejs.dev/config/
export default defineConfig({
plugins: [
react(),
removeConsole() // <-- here we go!
],
})
The Plugin File
Let’s take a look at the general structure of a plugin file then simply jump right in and write the utility function that will find and remove the console log with regex.
import type { PluginOption } from "vite";
interface Options {
includes: string[] | undefined;
external: string[] | undefined;
custom: string[] | undefined;
}
export default function myPluginName(
options: Partial<Options> = {}
): PluginOption {
return {
name: "vite:my-plugin-name",
apply: "build",
enforce: "post",
transform(_source: string, id: string) {
return {
code: _source,
map: null
};
}
};
}
export type { PluginOption };
As you can see above we have the imports of the plugin, mostly types, we build the function that return an object with few plugin related information and we use something called “transform” in vite, in there we’ll modify the code as we wish.
Here is the utility function that will help us remove the actual console, it will receive a minifiedCode which is a string which is the actual code and make the modification we want and return a new string:
// utility function
function removeConsoleLogs(minifiedCode:string) {
const consoleLogRegex = /console\.log\s*\(\s*("[^"]*"|'[^']*')\s*\)[,;]?/g;
return minifiedCode.replace(consoleLogRegex, '');
}
now we want to change the transform and apply the function that remove the console logs:
transform(_source: string, id: string) {
return {
code: removeConsoleLogs(_source),
map: null
};
}
The full plugin code:
// src/vite-plugins/vite-plugin-remove-console.ts
import type { PluginOption } from "vite";
interface Options {
includes: string[] | undefined;
external: string[] | undefined;
custom: string[] | undefined;
}
// utility function
function removeConsoleLogs(minifiedCode:string) {
const consoleLogRegex = /console\.log\s*\(\s*("[^"]*"|'[^']*')\s*\)[,;]?/g;
return minifiedCode.replace(consoleLogRegex, '');
}
export default function removeConsole(
options: Partial<Options> = {}
): PluginOption {
return {
name: "vite:remove-console",
apply: "build",
enforce: "post",
transform(_source: string, id: string) {
return {
code: removeConsoleLogs(_source),
map: null
};
}
};
}
export type { PluginOption };
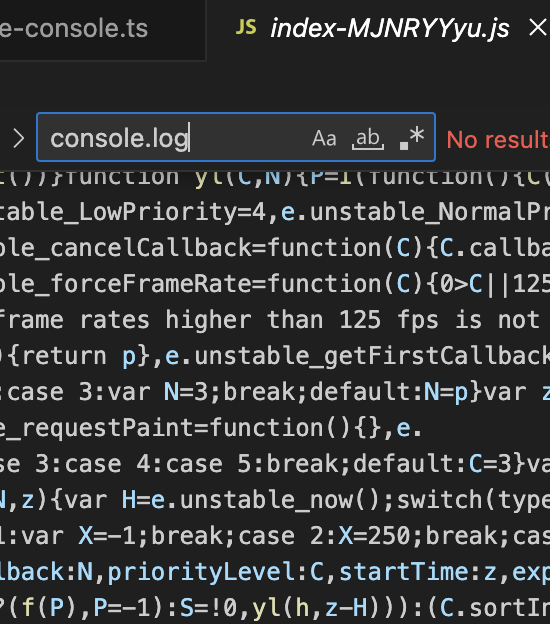
To put it to a test just run “yarn build” and check the folder “dist” with the newly generated index file with the new hash! and thats it, you have now learn where you can modify any code that go throughout your code base.
If you want me to send you git repo let me know in comments or in contact page.
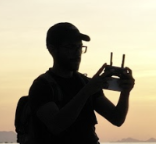
Lior Amsalem embarked on his software engineering journey in the early 2000s, delving into Pascal with a keen interest in creating, developing, and bringing new innovations to life. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply