Javascript intervals help us to manage actions that take time or perform behaviour that needs to be executed at a later point in time. Here’s a basic example of a javascript interval for a countdown clock.
let seconds = 0;
const intervalID = setInterval(() => {
seconds++;
console.log(‘seconds:’, seconds)
}, 1000);
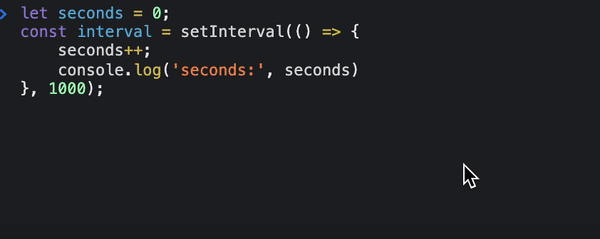
In the above example we define “seconds” variable than we bump that variable every 1000ms (which is 1 second). Then we log it to the console to see if it works good. If we’d want to stop the interval we’ll use clearInterval with the intervalID variable.
Difference between Intervals and setTimeout

How To, With React and Hooks?
Since the nature of the interval code is async, we’d want to use react code that supports side effects. In this case we are going to use useEffect. useEffect is meant for any side effects in react, data fetching, dom manipulation or subscription.
const [seconds, setSeconds] = useState(60);
useEffect(() => {
const interval = setInterval(() => {
setSeconds(seconds => seconds — 1);
}, 1000);
if(seconds <= 0) {
clearInterval(interval);
}
return () => clearInterval(interval);
}, [seconds]);
In the above example we count from 60 to 0. And we use useEffect to manage the async nature of intervals. We also keep a const of the setInterval so we could use clearInterval later. Note that we also set seconds as a dependency for useEffect so whenever the number changes it will continue to run the useEffect and if the number is below 0, we’ll clear the interval.
Important
Remember to clean up any side effect that can be cleaned to prevent memory leaks and apps that will run indefinitely and crash. In the Interval case use clearInterval.
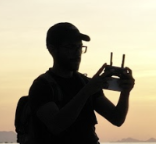
Lior Amsalem embarked on his software engineering journey in the early 2000s, Diving into Pascal with a keen interest in creating, developing, and working on new technologies. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops, nextjs, nodejs, mongodb, mysql and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply