Fine-grained reactivity refers to a programming paradigm or approach where updates to the user interface (UI) are handled at a very granular level, typically down to individual data changes. In the context of frontend development, this concept is often associated with libraries or frameworks that prioritize performance and efficiency by minimizing unnecessary re-renders of components.
In traditional virtual DOM-based frameworks like React, when the state of a component changes, the entire component is typically re-rendered. This approach can be inefficient, especially in large applications with complex UIs, as it can lead to unnecessary re-renders of components that are not affected by the state change.
Fine-grained reactivity addresses this issue by intelligently tracking dependencies between data and UI elements, ensuring that only the parts of the UI that are directly affected by state changes are updated. This means that if a specific piece of data changes, only the corresponding UI elements that depend on that data will be re-rendered, while other unaffected parts of the UI remain unchanged.
Libraries and frameworks that implement fine-grained reactivity often provide mechanisms for efficiently tracking dependencies and updating the UI in response to changes. This can involve techniques such as data binding, observables, or reactive programming patterns.
The benefits of fine-grained reactivity include improved performance, reduced rendering overhead, and a more responsive user experience, particularly in applications with dynamic content or frequent data updates. By minimizing unnecessary re-renders and optimizing UI updates at a granular level, developers can create faster and more efficient web applications that deliver a seamless user experience.
Code Example
To better understand fine-grained reactivity here’s a simple code example written in solidjs:
import { createSignal } from 'solid-js';
function Counter() {
const [count, setCount] = createSignal(0);
function increment() {
setCount(count() + 1); // Update the count state
}
return (
<div>
<h2>Count: {count()}</h2>
<button onClick={increment}>Increment</button>
</div>
);
}
function App() {
return (
<div>
<h1>Fine-Grained Reactivity in SolidJS</h1>
<Counter />
</div>
);
}
export default App;
in the above example, we use “createSignal”. In this example, we have a Counter
component that displays a count value and a button to increment it. We’re using the createSignal
function from SolidJS to create a reactive signal count
, which holds the current count value.
When the increment
function is called, it updates the count
signal by fetching the current value using count()
and then incrementing it by one. Because count
is a reactive signal, any component that depends on it will automatically re-render when its value changes.
This demonstrates fine-grained reactivity in SolidJS, where only the part of the UI displaying the count value will be updated when it changes, rather than re-rendering the entire component. This approach ensures efficient updates and optimal performance, especially in larger applications with more complex UIs.
What Is Create Signal
In SolidJS, the createSignal
function is a core part of its reactive programming model. It’s used to create a reactive signal, which is essentially a piece of state that can be observed and automatically updated whenever its value changes.
Here’s a breakdown of what createSignal
does:
- Signal Creation: When you call
createSignal(initialValue)
, it creates a new reactive signal with an initial value. In the example provided,const [count, setCount] = createSignal(0);
creates a signal namedcount
with an initial value of0
. - Signal Value: The signal created by
createSignal
consists of two parts: the getter function and the setter function. The getter function (count()
in our example) retrieves the current value of the signal. - Reactivity: Whenever the value of the signal changes, any part of the UI that depends on that signal will automatically re-render to reflect the updated value. This is the essence of fine-grained reactivity in SolidJS.
- Updating the Signal: The setter function (
setCount
in our example) is used to update the value of the signal. When you callsetCount(newValue)
, it updates the value of thecount
signal tonewValue
, triggering any dependent UI elements to re-render.
Overall, createSignal
is a fundamental building block in SolidJS for managing state and enabling reactive updates in components. It facilitates a declarative and efficient approach to building user interfaces, where changes in state seamlessly propagate through the UI, resulting in a more responsive and interactive user experience.
Fine-grained Reactivity VS Virtual DOM Performance
Below you’ll see few common rendering cases, create new rows, partial update, remove row an more, and the average rendering time it took each library abd each approach to completely render it’s UI view.
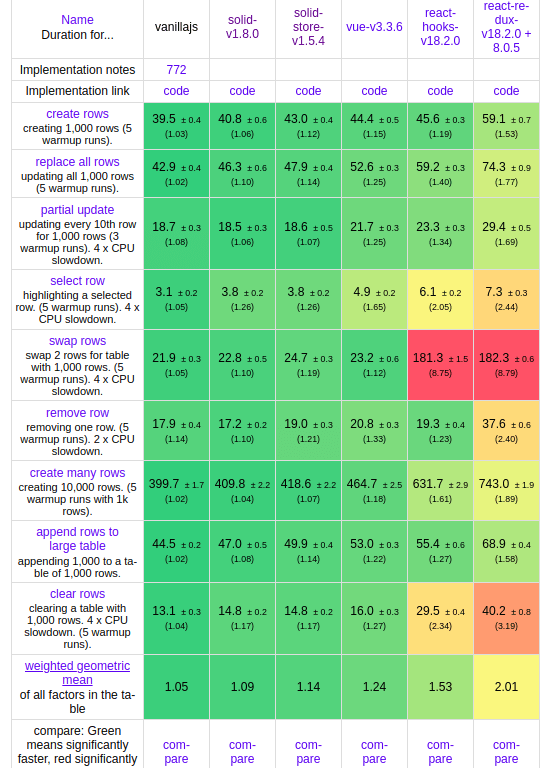
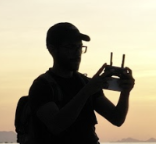
Lior Amsalem embarked on his software engineering journey in the early 2000s, delving into Pascal with a keen interest in creating, developing, and bringing new innovations to life. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply