In React.js, fragments provide a way to group multiple elements without adding extra nodes to the DOM. They are especially useful when you need to return multiple elements from a component’s render method. Fragments were introduced in React 16.2 as a lightweight way to group elements.
Using Fragments in JSX
Here’s an example of using fragments in a functional component:
import React from 'react';
const App = () => {
return (
<>
<h1>Hello, World!</h1>
<p>Welcome to my React blog.</p>
</>
);
};
export default App;
In this example, the ‘<>’ and ‘</>’are shorthand syntax for fragments. They allow you to group the ‘<h1>’ and ‘<p>’ elements without adding a wrapper ‘<div>’ or any other element to the DOM.
Key Benefits of Fragments
- Cleaner JSX: Fragments help to keep your JSX cleaner by avoiding unnecessary wrapper elements.
- Improved Performance: Since fragments don’t add extra nodes to the DOM, they can lead to better performance, especially in large applications.
- Avoiding Styling Issues: Fragments can help avoid CSS styling issues that may arise from adding extra wrapper elements.
Fragments with Keys
When you need to map over a list of elements and return them as fragments, you can use the key attribute to provide a unique identifier for each fragment:
import React from 'react';
const App = () => {
const items = ['Apple', 'Banana', 'Orange'];
return (
<>
{items.map((item, index) => (
<React.Fragment key={index}>
<p>{item}</p>
</React.Fragment>
))}
</>
);
};
export default App;
In this example, each ‘<React.Fragment>’ element is given a unique ‘key’ based on its ‘index’ in the ‘items’ array. This helps React identify each fragment and efficiently update the DOM when the list changes.
Conclusion
Fragments provide a convenient way to group elements in React.js without introducing extra nodes to the DOM. They help keep your JSX clean and improve performance, making them a valuable tool in your React component toolbox.
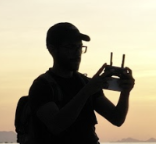
Lior Amsalem embarked on his software engineering journey in the early 2000s, Diving into Pascal with a keen interest in creating, developing, and working on new technologies. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops, nextjs, nodejs, mongodb, mysql and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply