Next.js, a popular React framework for building modern web applications, offers a wide range of powerful features like server-side rendering, static site generation, and API routes. One of the key tools for adding advanced functionality and control over requests in Next.js is Middleware. In this article, we’ll explore what middleware is in the context of Next.js, how it works, and how to implement it in your Next.js applications.
What is Middleware in Next.js?
Middleware in Next.js is a way to run custom code before a request is completed, providing an opportunity to handle tasks like authentication, redirects, and more before the request reaches the intended page or API route.
Middleware runs on the Edge in Next.js, meaning it executes on Vercel’s Edge Network (if deployed there), providing low-latency responses to users globally. This allows you to inspect incoming requests and modify the response accordingly, all without needing a full server.
When to Use Middleware?
Middleware is useful for scenarios where you need to:
- Authenticate users: Ensure users are logged in before allowing access to certain pages.
- Handle redirects: Redirect users to different routes based on conditions.
- Modify headers: Add, remove, or modify HTTP headers on requests and responses.
- Rate limiting: Control the number of requests made to certain endpoints.
- Logging and analytics: Track user requests for performance monitoring or analytics.
How Next.js Middleware Works
Middleware in Next.js works by intercepting a request and allows you to alter the response, reroute the request, or even abort it. You define middleware in a special file: middleware.ts or middleware.js located in the root of your Next.js project.
Here’s a simple structure:
├── pages/
│ ├── index.js
│ ├── about.js
├── public/
├── styles/
├── middleware.js
Next.js automatically picks up this file and executes it for all incoming requests to your site.
Anatomy of Middleware
A Next.js middleware function is a simple function that exports a NextResponse
or NextRequest
object:
import { NextResponse } from 'next/server';
export function middleware(req) {
// Custom logic here
return NextResponse.next();
}
Here, the NextResponse.next()
method passes the request through to the next handler or the page itself.
Example: Using Middleware to Redirect Unauthenticated Users
Let’s consider a practical example where you want to redirect users who are not logged in to a login page.
import { NextResponse } from 'next/server';
export function middleware(req) {
const token = req.cookies.get('token');
if (!token) {
return NextResponse.redirect(new URL('/login', req.url));
}
return NextResponse.next();
}
This is useful when you need to provide extra information in your responses, such as tracking information or additional security headers.
Middleware and Dynamic Routes
Middleware works seamlessly with dynamic routes. For instance, if you have a dynamic route like /user/[id]
, you can apply middleware to inspect and handle different id
values accordingly.
export function middleware(req) {
const { pathname } = req.nextUrl;
const userId = pathname.split('/')[2]; // Extract the dynamic [id] from /user/[id]
// Add logic based on userId, e.g., load user-specific data or apply specific logic
if (userId === '123') {
return NextResponse.rewrite(new URL('/special-page', req.url));
}
return NextResponse.next();
}
Middleware in API Routes
Middleware in Next.js can also be used with API routes to control access, authenticate users, or modify the request before hitting the API.
For example, to add basic rate-limiting on an API route:
let count = 0;
export function middleware(req) {
count++;
if (count > 10) {
return new NextResponse('Rate limit exceeded', { status: 429 });
}
return NextResponse.next();
}
This counts the number of requests and blocks any after the tenth one, returning a 429 Rate Limit Exceeded
response.
Limitations and Considerations
While middleware is a powerful tool, there are a few limitations and considerations to keep in mind:
- No access to the response body: Middleware can modify headers and status codes, but it can’t modify the actual content of the response body.
- Edge runtime: Middleware runs in the Edge environment, which is optimized for performance but lacks certain Node.js APIs, such as
fs
(file system access). You’ll need to work with Web APIs instead. - Caching: Middleware can interact with caching strategies, which might affect how often your middleware is executed or whether your dynamic logic is appropriately reflected in responses.
Conclusion
Next.js Middleware is a powerful feature that provides fine-grained control over requests, enabling tasks such as authentication, redirects, and header manipulation. By intercepting requests early, middleware opens the door for performance optimizations and enhanced security.
Whether you’re building complex user authentication flows or setting up global logging mechanisms, Next.js middleware is a versatile tool to incorporate into your applications.
Experiment with different use cases, and enjoy the flexibility it brings to your Next.js projects!
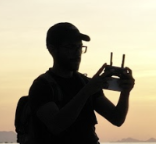
Lior Amsalem embarked on his software engineering journey in the early 2000s, Diving into Pascal with a keen interest in creating, developing, and working on new technologies. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops, nextjs, nodejs, mongodb, mysql and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply