In many cases when we write JSX code, HTML inside a react component and we’ll be using typescript. We’ll find ourself interacting with build in events of the browser. one of those events is known as “HTMLSelectElement” or the event that occurs when a user click on an element inside “option” and “Select” html tags. When user interact with those items, The browser fires event and we want to catch that event (to see what the user pick from the list of options) and perform actions accordingly.
Before you jump in, you can also learn how to code with gpteach.us AI that pitch you coding tasks to gain your 10,000 hours code-writing-skills and land your dream job.
In order to make the event itself accessible via Typescript here’s What we’ll do:
- We’ll import changeEvent from react
- We’ll use it to assign to the change event function
- Than we’ll use “HTMLSelectElement” to define the type of the change event, which is a select HTML element.
onChange Event select/option
For a <select>
element, the onChange
event is triggered when the user selects a different option from the dropdown. In React with TypeScript, you can type the event handler to ensure that it specifically handles events from a <select>
element.
import React, { ChangeEvent } from 'react';
// Define your component
const YourComponent: React.FC = () => {
// Define the event handler function
const handleMenuOnChange = (e: ChangeEvent<HTMLSelectElement>) => { // <----- here we assign event to ChangeEvent
// Your logic here
console.log(e.target.value); // Example: Log the value of the selected option
};
// Render your component with the event handler attached
return (
<select onChange={handleMenuOnChange}>
{/* Your select options */}
<option value="1">one</option>
<option value="2">two</option>
</select>
);
};
export default YourComponent;
In this example:
- We import
ChangeEvent
from'react'
, which represents the type of the change event. - The
handleApartmentMenuOnChange
function takes an event object of typeChangeEvent<HTMLSelectElement>
, whereHTMLSelectElement
represents the type of the HTML select element. - Within the event handler, you can access properties of the event object like
e.target.value
to get the value of the selected option.
Breakdown:
- Event Type:
- The event passed to the
onChange
handler is of typeReact.ChangeEvent<HTMLSelectElement>
. This ensures that TypeScript knows the event is specifically associated with a<select>
element, enabling type safety. React.ChangeEvent<HTMLSelectElement>
tells TypeScript that this is an event from a<select>
HTML element. This is important for ensuring that thetarget
property inside the event corresponds to the right HTML element type (HTMLSelectElement
).
- The event passed to the
- Accessing the Selected Value:
e.target.value
retrieves the currently selected value from the dropdown. Thetarget
refers to the<select>
element that triggered the event, andvalue
is the value of the currently selected<option>
.- This value is always a string, even if the options are numbers or other data types.
- Handler Function:
- The
handleSelectChange
function is the event handler that gets invoked every time the user selects a new option from the dropdown. - Inside this function,
e.target.value
is captured asselectedValue
, which holds the value of the currently selected option. console.log(selectedValue)
is used to print the selected value to the console for debugging purposes.
- The
- JSX Usage:
The<select>
element uses theonChange
attribute to specify thehandleSelectChange
function as the event handler. Whenever the user picks a different option, this function is executed.Inside the<select>
tag, the<option>
elements represent the choices available to the user. Each<option>
has avalue
attribute, which corresponds to the value that will be passed toe.target.value
when that option is selected.
Example in Action:
- Initially, the user sees the dropdown with “Option 1” and “Option 2.”
- If the user selects “Option 2”, the
onChange
event is triggered, andhandleSelectChange
is called. - Inside the event handler,
e.target.value
will be"option2"
, which is the value associated with the selected option.
In this example, onChange
is used to handle changes in the <select>
dropdown, and TypeScript’s React.ChangeEvent<HTMLSelectElement>
type ensures the event handler works specifically with a <select>
element. The value
of the selected option is accessed via e.target.value
and can be used within the handler function. This guarantees type safety and avoids potential runtime issues related to incorrect element handling.
onChange Input of checkbox
For the checkbox (<input type="checkbox">
), the onChange
event is triggered whenever the user checks or unchecks the box. In React with TypeScript, you need to properly type the event handler to ensure type safety. Here’s how it works:
const handleCheckboxChange = (e: React.ChangeEvent<HTMLInputElement>) => {
const isChecked = e.target.checked;
console.log(isChecked);
};
return <input type="checkbox" onChange={handleCheckboxChange} />;
Breakdown:
- Event Type:
The event passed to theonChange
handler is aReact.ChangeEvent<HTMLInputElement>
. In TypeScript, this ensures that the event is specifically tied to an HTML<input>
element. This is important becauseonChange
events behave differently for various form elements.React.ChangeEvent<HTMLInputElement>
is the type that tells TypeScript the event is coming from an<input>
element (specifically, in this case, a checkbox).
- Accessing the Checkbox State:
e.target.checked
is the property used to determine whether the checkbox is currently checked or not.checked
is a boolean value (true
if the checkbox is checked,false
if it is unchecked).
- Handler Function:
- The
handleCheckboxChange
function captures the event when the checkbox state changes and logs the currentchecked
state. isChecked
stores the value ofe.target.checked
.
- The
- Usage:
When a user checks or unchecks the checkbox, theonChange
handler is invoked, and it logs the new state (true
orfalse
) of the checkbox.
The key point here is that for checkboxes, unlike text inputs, the value you’re interested in is the checked
state, which is a boolean. TypeScript ensures that the checked
property is correctly typed, giving you proper type safety when working with form inputs in React.
onChange Event Type for checkbox
When using a checkbox in HTML (), the onChange event is triggered whenever the user checks or unchecks the box. In React with TypeScript, we need to ensure that the event handler is properly typed to handle a checkbox and retrieve its checked state, which is a boolean value.
const handleCheckboxChange = (e: React.ChangeEvent<HTMLInputElement>) => {
const isChecked = e.target.checked;
console.log(isChecked);
};
return <input type="checkbox" onChange={handleCheckboxChange} />;
Breakdown:
- Event Type:
- The event passed to the
onChange
handler is of typeReact.ChangeEvent<HTMLInputElement>
. This ensures that the event is specifically associated with an<input>
element, particularly one of typecheckbox
. React.ChangeEvent<HTMLInputElement>
is used to tell TypeScript that the event is coming from an<input>
element. Since checkboxes are handled differently than other types of inputs (such as text fields), we want TypeScript to know it’s working with the right element type.
- The event passed to the
- Accessing the Checkbox State (
checked
):e.target.checked
is used to retrieve the current state of the checkbox (whether it is checked or unchecked).checked
is a boolean property:true
if the checkbox is checked andfalse
if it is unchecked.
- This is different from other form elements like text inputs, where you’d access
e.target.value
. For checkboxes,checked
is the relevant property.
- Handler Function:
- The
handleCheckboxChange
function is triggered whenever the checkbox is toggled (checked or unchecked). - Inside the function,
e.target.checked
is used to capture the checkbox’s state (true
orfalse
) and assign it toisChecked
. console.log(isChecked)
is used to log the current state of the checkbox to the console for debugging or other purposes.
- The
- JSX Usage:
- The
<input>
element is of typecheckbox
. TheonChange
attribute attaches thehandleCheckboxChange
function to handle the event when the checkbox is toggled. - Every time the user clicks on the checkbox to check or uncheck it, the
onChange
event is triggered, and thehandleCheckboxChange
function is executed.
- The
Example in Action:
- The user sees a checkbox on the screen.
- If the checkbox is checked, the
onChange
event is triggered, andhandleCheckboxChange
is called. - Inside the handler,
e.target.checked
will betrue
because the checkbox is now checked, and it is printed in the console. - If the checkbox is unchecked,
e.target.checked
will befalse
, and it will logfalse
to the console.
Key Points:
- Checkboxes Use the
checked
Property: Unlike other input types, where you deal with thevalue
property, checkboxes have achecked
property that is a boolean. - Event Type in TypeScript:
React.ChangeEvent<HTMLInputElement>
ensures the event handler is typed correctly to work with a checkbox input element, making the code type-safe. - Boolean Result: Since a checkbox can only be either checked (
true
) or unchecked (false
), this simple binary result is what you work with when handling checkboxes.
In the case of a checkbox, the onChange
event works with the checked
property, which returns a boolean (true
or false
). By using React.ChangeEvent<HTMLInputElement>
in TypeScript, you ensure the event handler is correctly typed for an <input>
of type checkbox
, which gives you type safety and avoids potential errors when working with checkboxes in a React app.
onChange Event input radio
When using radio buttons in HTML (), the onChange event is triggered when a user selects a radio button from a group. In React with TypeScript, it is essential to properly type the event handler to capture the selected radio button’s value.
const handleRadioChange = (e: React.ChangeEvent<HTMLInputElement>) => {
const selectedRadioValue = e.target.value;
console.log(selectedRadioValue);
};
return (
<>
<input type="radio" value="option1" name="radioGroup" onChange={handleRadioChange} />
<input type="radio" value="option2" name="radioGroup" onChange={handleRadioChange} />
</>
);
Breakdown:
- Event Type:
- The event parameter passed to the
onChange
handler is typed asReact.ChangeEvent<HTMLInputElement>
. This ensures TypeScript understands that the event originates from an<input>
element. - Using
React.ChangeEvent<HTMLInputElement>
is crucial for type safety, as it specifies that the event pertains to a radio button input.
- The event parameter passed to the
- Accessing the Selected Radio Button’s Value:
e.target.value
retrieves the value of the selected radio button from the event target (the specific radio button that triggered the change).- Each radio button in a group should have a
value
attribute that specifies what value to return when that button is selected.
- Handler Function:
- The
handleRadioChange
function is called whenever a radio button is selected. - Inside this function,
e.target.value
is used to capture the value of the selected radio button and assign it to the variableselectedRadioValue
. console.log(selectedRadioValue)
prints the value to the console, allowing you to see which radio button was selected.
- The
- JSX Usage:
- The radio buttons are represented by two
<input>
elements, both of typeradio
. - Each radio button has the same
name
attribute ("radioGroup"
), which groups them together. This means only one of them can be selected at a time. - The
onChange
attribute of each radio button is set to call thehandleRadioChange
function whenever the user selects a button.
- The radio buttons are represented by two
Example in Action:
- The user sees two radio buttons labeled “Option 1” and “Option 2.”
- When the user clicks on “Option 1,” the
onChange
event is triggered, invoking thehandleRadioChange
function. - Inside the handler,
e.target.value
will return"option1"
, which is printed to the console. - If the user later clicks on “Option 2,” the event will again trigger, and
e.target.value
will return"option2"
.
Key Points:
- Grouping Radio Buttons: All radio buttons in the same group should share the same
name
attribute. This allows only one button in that group to be selected at any time. - Value Property: Each radio button should have a
value
attribute to determine what value gets returned when that button is selected. - Event Type in TypeScript: By using
React.ChangeEvent<HTMLInputElement>
, you ensure that the event handler is correctly typed for an input of typeradio
, which enhances type safety.
In summary, handling the onChange
event for radio buttons in React with TypeScript involves using React.ChangeEvent<HTMLInputElement>
to ensure type safety. The value
of the selected radio button is accessed via e.target.value
, which provides information about the user’s selection. This setup allows you to efficiently manage and respond to user input when working with radio buttons in a React application.
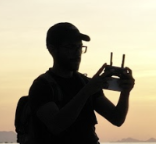
Lior Amsalem embarked on his software engineering journey in the early 2000s, Diving into Pascal with a keen interest in creating, developing, and working on new technologies. Transitioning from his early teenage years as a freelancer, Lior dedicated countless hours to expanding his knowledge within the software engineering domain. He immersed himself in learning new development languages and technologies such as JavaScript, React, backend, frontend, devops, nextjs, nodejs, mongodb, mysql and all together end to end development, while also gaining insights into business development and idea implementation.
Through his blog, Lior aims to share his interests and entrepreneurial journey, driven by a desire for independence and freedom from traditional 9-5 work constraints.
Leave a Reply